配置文件alipay_config.php:
return [
//'配置项'=>'配置值'
//应用ID,您的APPID。
'app_id' => "",
//商户私钥,您的原始格式RSA私钥 生成方法https://docs.open.alipay.com/291/105971
'merchant_private_key' => "",
//异步通知地址
'notify_url' => "",
//同步跳转
'return_url' => "",
//编码格式
'charset' => "UTF-8",
//签名方式
'sign_type'=>"RSA2",
//支付宝网关,正式环境https://openapi.alipay.com/gateway.do?
'gatewayUrl' => "https://openapi.alipaydev.com/gateway.do?",//沙箱
//支付宝公钥,查看地址:https://openhome.alipay.com/platform/keyManage.htm 对应APPID下的支付宝公钥。
'alipay_public_key' => "",
];
支付类AliPay.php:
class AliPay{
public $config=[];
//私钥文件路径
private $rsaPrivateKeyFilePath='';
public $rsaPrivateKey=''; //商户私钥
public $fileCharset='utf-8';
//销售产品码 H5(QUICK_WAP_WAY) PC支付(FAST_INSTANT_TRADE_PAY)
public $product_code='FAST_INSTANT_TRADE_PAY';
//接口名称 H5(alipay.trade.wap.pay) PC支付(alipay.trade.page.pay)
public $method='alipay.trade.page.pay';
public function __construct($config=[]){
$include_conf=include_once 'alipay_config.php';
$this->config=array_merge($config,$include_conf);
$this->rsaPrivateKey=$this->config['merchant_private_key'];
$this->fileCharset=$this->config['charset'];
}
/**
* @date 2018-10-2
* Pc端支付
*/
public function PcPay($data=[]){
$data['product_code']='FAST_INSTANT_TRADE_PAY';
$this->method='alipay.trade.page.pay';
$re=$this->Pay($data);
return $re;
}
/**
* @date 2018-10-2
* 手机网站支付
*/
public function WapPay($data=[]){
$data['product_code']='QUICK_WAP_WAY';
$this->method='alipay.trade.wap.pay';
$re=$this->Pay($data);
return $re;
}
/**
* @date 2018-10-2
* 支付
*/
private function Pay($data=[]){
$json_data=json_encode($data);
$parm_data=array(
'app_id' => $this->config['app_id'],
'version' => '1.0',//固定值
'format' => 'JSON',//固定值
'sign_type' =>$this->config['sign_type'],
'method' =>$this->method,//固定值
'timestamp' => date('Y-m-d H:i:s'),
'notify_url' => $this->config['notify_url'],//回调地址
'return_url' => $this->config['return_url'],//同步跳转
'charset' => $this->config['charset'],//固定值
'biz_content' => $json_data
);
//待签名字符串
$str = $this->getSignContent($parm_data);
// echo $str;die;
$sign=$this->sign($str,'RSA2');
$url=$this->config['gatewayUrl'].$str.'&sign='.urlencode($sign);
return $url;
}
protected function sign($data, $signType = "RSA") {
if($this->checkEmpty($this->rsaPrivateKeyFilePath)){
$priKey=$this->rsaPrivateKey;
$res = "-----BEGIN RSA PRIVATE KEY-----\n" .
wordwrap($priKey, 64, "\n", true) .
"\n-----END RSA PRIVATE KEY-----";
}else {
$priKey = file_get_contents($this->rsaPrivateKeyFilePath);
$res = openssl_get_privatekey($priKey);
}
($res) or die('您使用的私钥格式错误,请检查RSA私钥配置');
if ("RSA2" == $signType) {
openssl_sign($data, $sign, $res, OPENSSL_ALGO_SHA256);
} else {
openssl_sign($data, $sign, $res);
}
if(!$this->checkEmpty($this->rsaPrivateKeyFilePath)){
openssl_free_key($res);
}
$sign = base64_encode($sign);
return $sign;
}
protected function getSignContent($params) {
ksort($params);
$stringToBeSigned = "";
$i = 0;
foreach ($params as $k => $v) {
if (false === $this->checkEmpty($v) && "@" != substr($v, 0, 1)) {
// 转换成目标字符集
$v = $this->characet($v, $this->fileCharset);
if ($i == 0) {
$stringToBeSigned .= "$k" . "=" . "$v";
} else {
$stringToBeSigned .= "&" . "$k" . "=" . "$v";
}
$i++;
}
}
unset ($k, $v);
return $stringToBeSigned;
}
/**
* 校验$value是否非空
* if not set ,return true;
* if is null , return true;
**/
protected function checkEmpty($value) {
if (!isset($value))
return true;
if ($value === null)
return true;
if (trim($value) === "")
return true;
return false;
}
/**
* 转换字符集编码
* @param $data
* @param $targetCharset
* @return string
*/
function characet($data, $targetCharset) {
if (!empty($data)) {
$fileType = $this->fileCharset;
if (strcasecmp($fileType, $targetCharset) != 0) {
$data = mb_convert_encoding($data, $targetCharset, $fileType);
//$data = iconv($fileType, $targetCharset.'//IGNORE', $data);
}
}
return $data;
}
}
调用示例index.php:
//电脑网站支付
function pc_pay(){
require_once 'AliPay.php';
$model=new AliPay();
$data=[
'subject'=>'测试订单',//商品的标题
'out_trade_no'=>time().uniqid(),//商户网站唯一订单号
'total_amount'=>'100',//订单总金额,单位为元
];
$url=$model->PcPay($data);
header("Location: {$url}");
};
//手机网站支付
function wap_pay(){
require_once 'AliPay.php';
$model=new AliPay();
$data=[
'subject'=>'测试订单',//商品的标题
'out_trade_no'=>time().uniqid(),//商户网站唯一订单号
'total_amount'=>'100',//订单总金额,单位为元
];
$url=$model->WapPay($data);
header("Location: {$url}");
}
//wap_pay();
pc_pay();
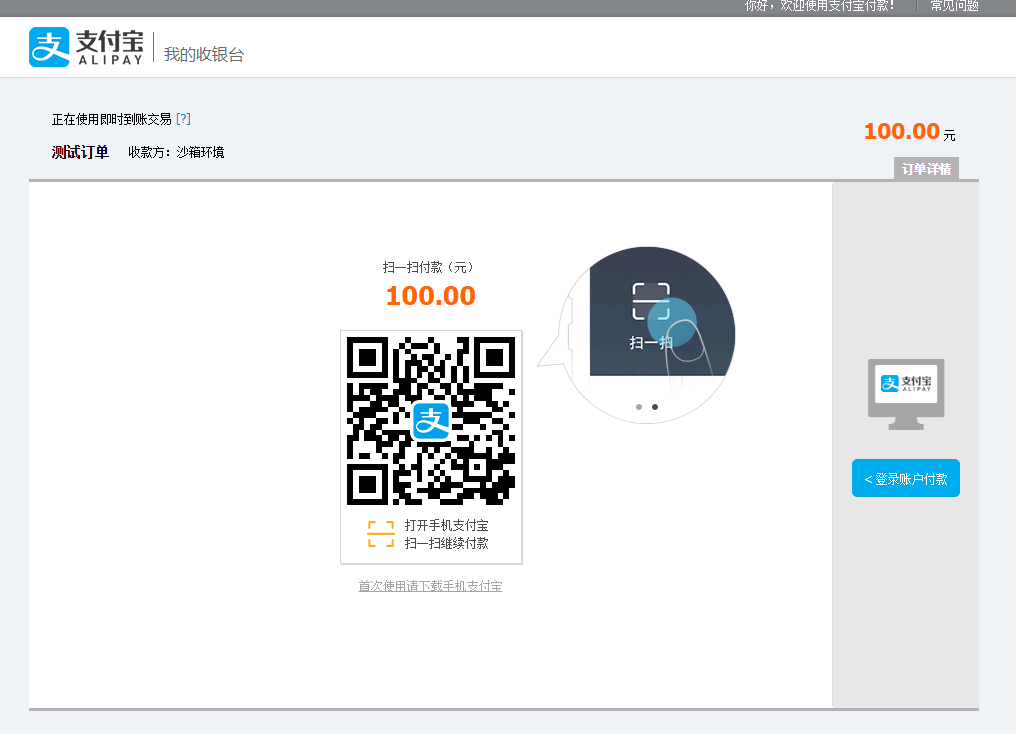